Make Azure Function in Local
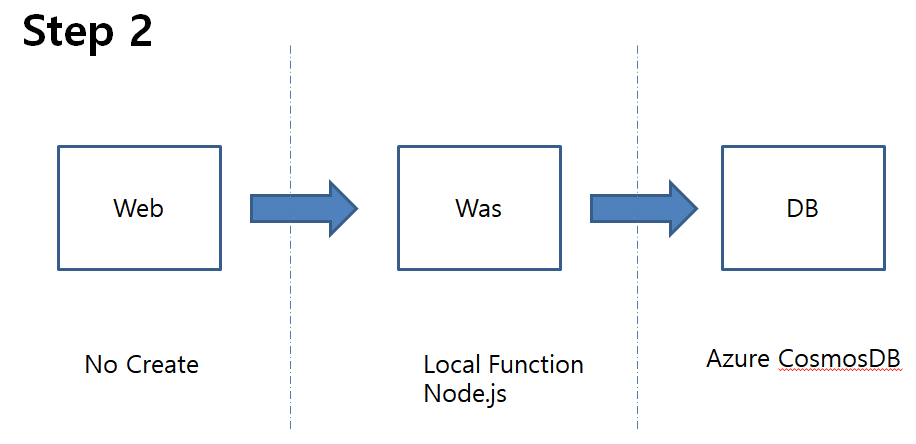
requirement
Install Node.js
Install Visual Studio Code.
Open the VS Code. i set the path C:\dev\blog-backend\
.
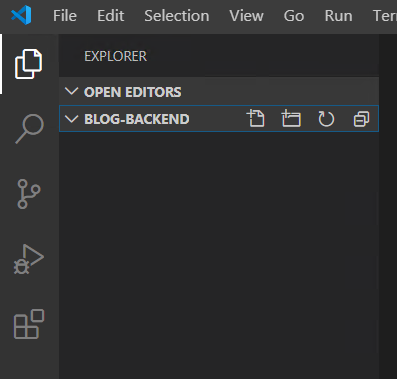
Install Azure Function in Extension.
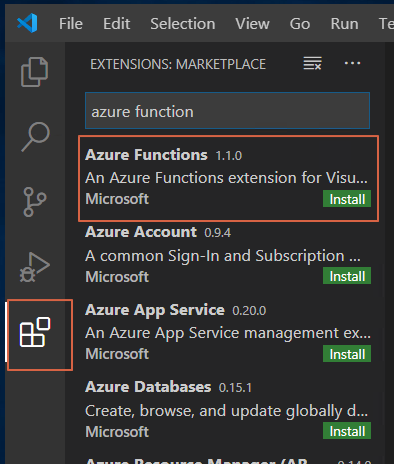
After install, you can show Azure Extension in left bar like picture.
And click Sign in to Azure… for connect your subscription.
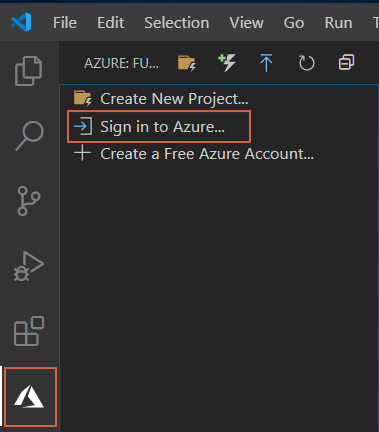
After Auth, i connected my subscription.
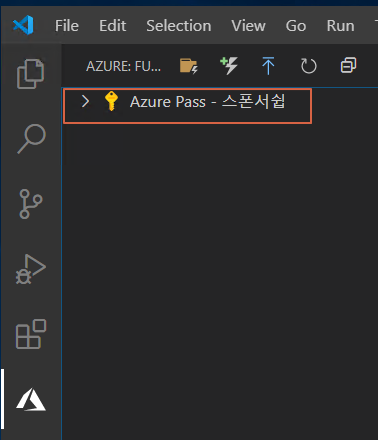
And Right Click, Click Create Function App in Azure
Then type name you want. i named user-blog_backend
and select language node.js 12
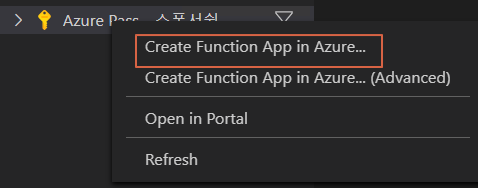
After a few minute, we check function app created under the picture.
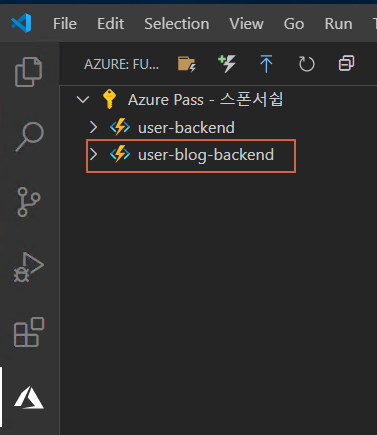
And select Azure function you created, Click icon Lightning.
And select javascript, HTTP trigger.
Type the name you want. i named create-user.
Then select anonymous.
Finally, create default code like this picture.
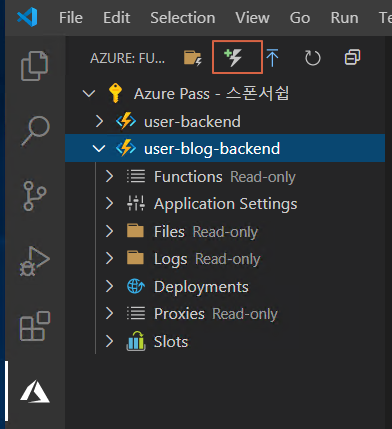
We execute this code using press F5.
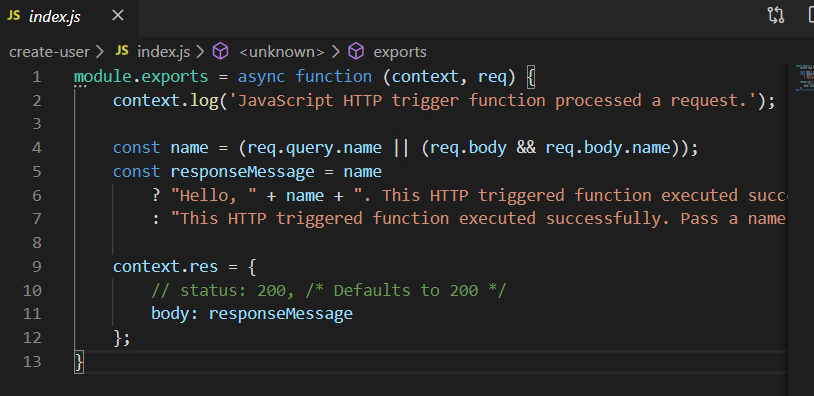
tTen logging endpoint in terminal.
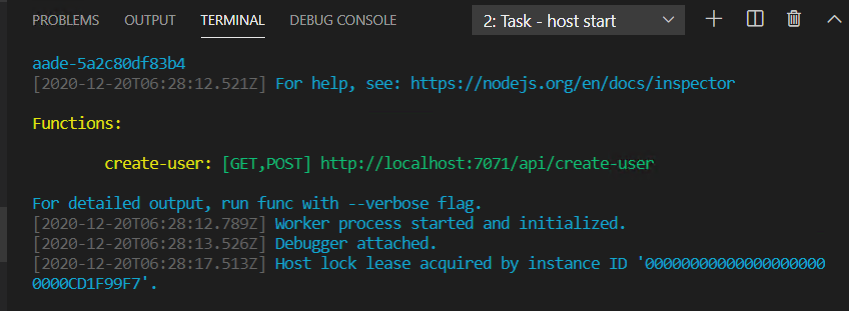
I try GET method using browser. it’s working well.
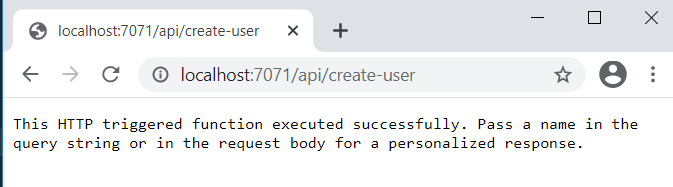
And we change code, add file.create-user/index.js
1 | module.exports = async function (context, req) { |
Create folder and file model/user_model.js
.
1 | var mongoose = require('mongoose'); |
Create file Config.js
in root path.
You need to refer cosmosDB connection_string in portal.
1 | module.exports = { |
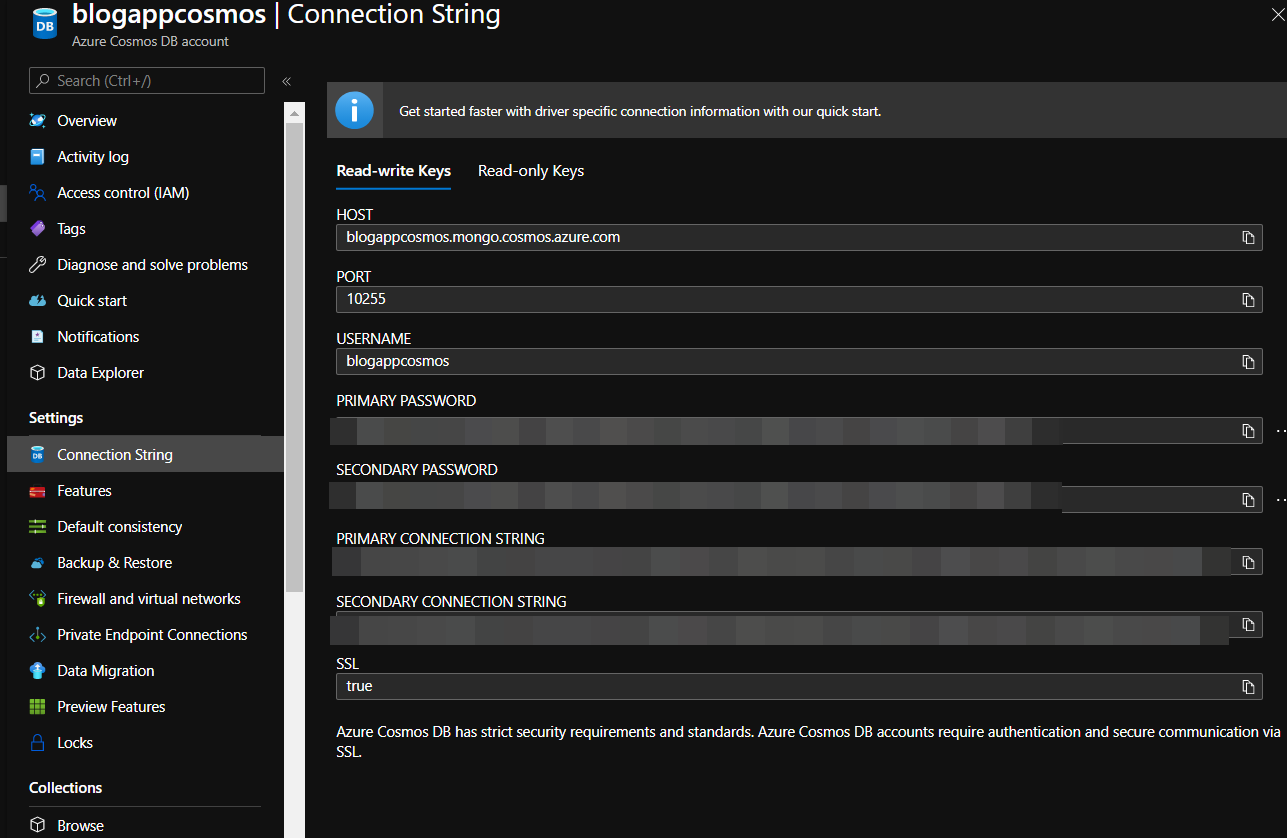
And we add mongoose module.
Execute cmd in terminal.
1 | npm install mongoose@5.11.8 --save |
After install well, execute function press F5 in index.js.
And test the function like this.
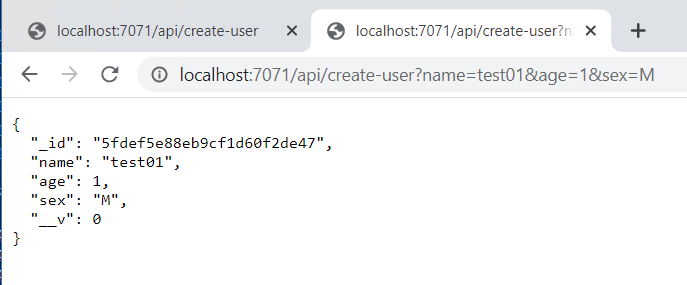
And we check the result in portal.
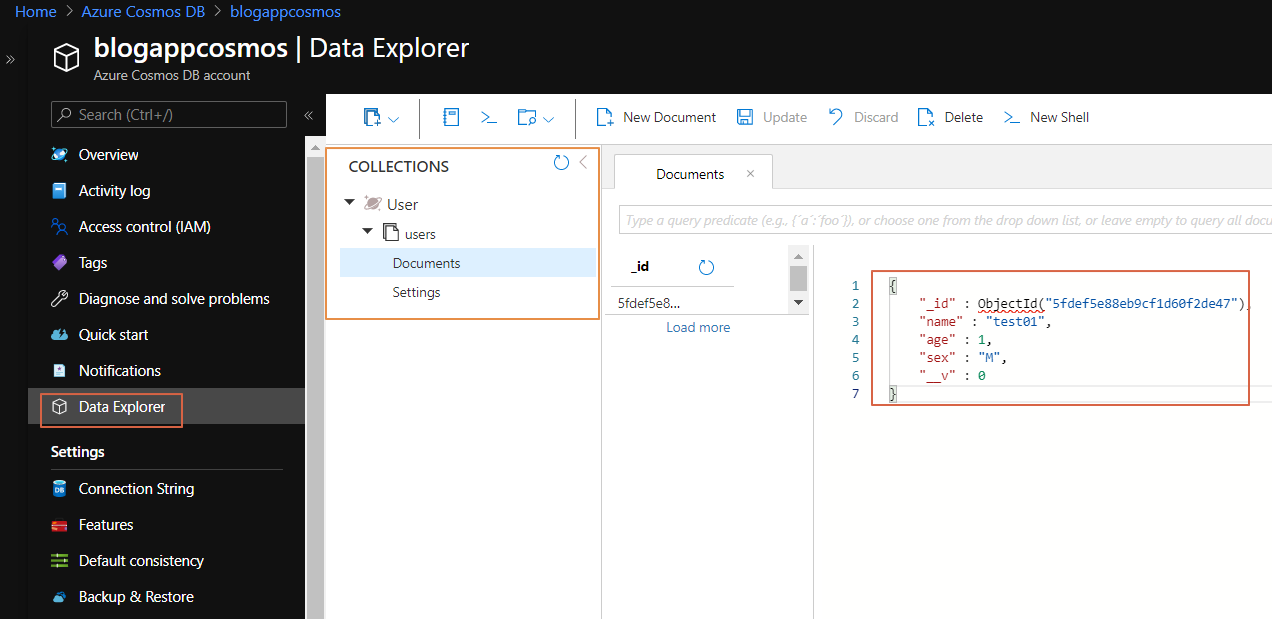
And i made two data test02
, test03
using exeucte API .
get user data
We create additional function named find-user.
and change codefind-user\index.js
1 | module.exports = async function (context, req) { |
Then, test the function like this.
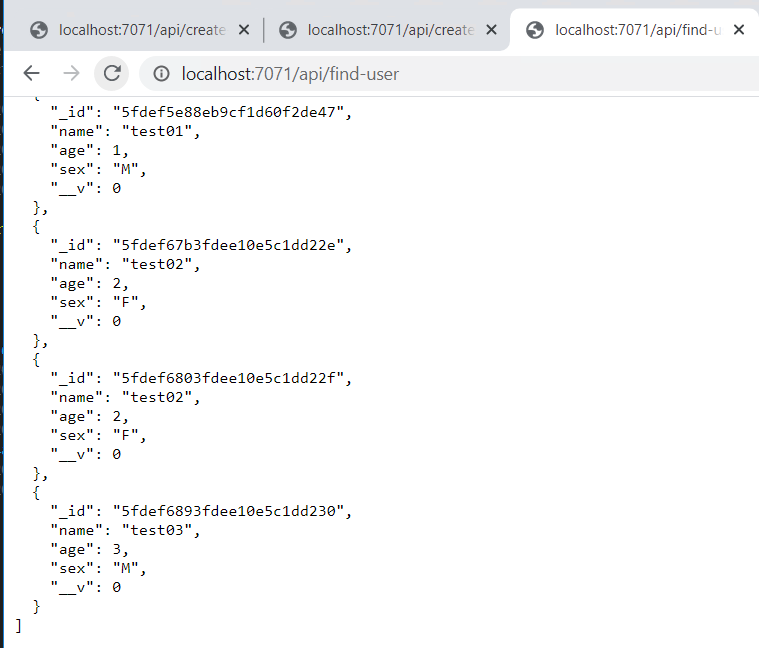
We finish make Azure Function App in local.
Good job!!
Related Posts
Azure app architecure - SummaryAzure app architecure - step01
Azure app architecure - step03
Azure app architecure - step04
Azure app architecure - step05